While preparing for my talk at Gotham Phyiscs X ML I made my first word cloud pun using Andreas Mueller's word_cloud tool.
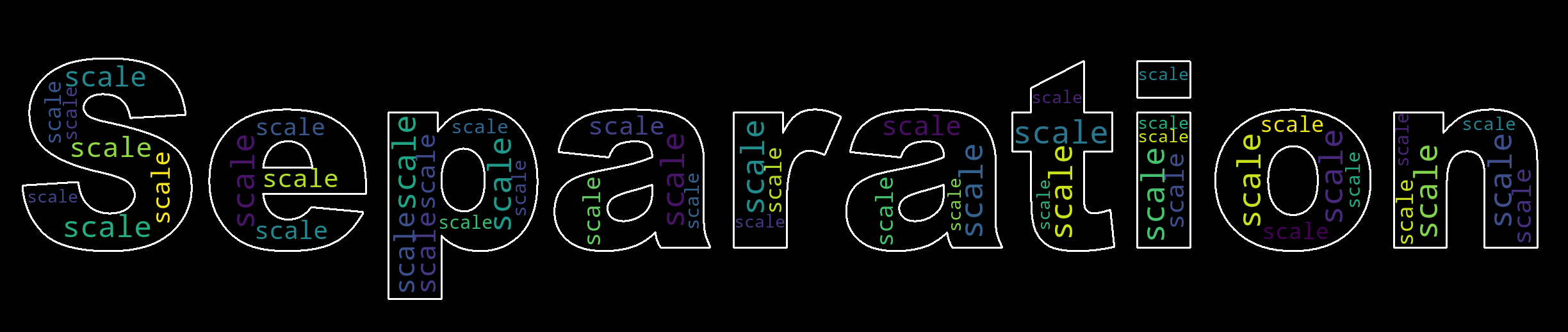
Code:
In [20]:
import matplotlib
In [21]:
#!/usr/bin/env python
"""
Masked wordcloud
================
Using a mask you can generate wordclouds in arbitrary shapes.
"""
from os import path
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
import os
from wordcloud import WordCloud, STOPWORDS
# get data directory (using getcwd() is needed to support running example in generated IPython notebook)
d = path.dirname(__file__) if "__file__" in locals() else os.getcwd()
# Read the whole text.
#text = open(path.join(d, 'alice.txt')).read()
text = "scale "*100
# read the mask image
# taken from
# http://www.stencilry.org/stencils/movies/alice%20in%20wonderland/255fk.jpg
alice_mask = np.array(Image.open(path.join(d, "separation.png")))
#stopwords = set(STOPWORDS)
#stopwords.add("said")
wc = WordCloud(background_color="white", max_words=200, repeat=True, mask=alice_mask,
stopwords=stopwords, contour_width=3, contour_color='steelblue')
wc = WordCloud(background_color="black", max_words=50, repeat=True, mask=alice_mask,
stopwords=stopwords, contour_width=3, contour_color='white')
# generate word cloud
wc.generate(text)
# store to file
wc.to_file(path.join(d, "alice.png"))
# show
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.figure()
plt.imshow(alice_mask, cmap=plt.cm.gray, interpolation='bilinear')
plt.axis("off")
plt.show()
Comments
comments powered by Disqus